Java 8 for Mac OS and writing a Hello World using Lambda ⮐
Follow the instruction to download java-8 lambda featured JDK & JRE.
-
Get the java 8 lambda package
-
Unzip and put it in some folder that you wanted or preferably at
/System/Library/Java/java-8-lambda
-
Open terminal and set your
JAVA_HOME
to point to your path and now execute thejava
&javac
commands to see if the libraries are good.
$export JAVA_HOME=/System/Library/Java/java-8-lambda
$java -version
openjdk version "1.8.0-ea"
OpenJDK Runtime Environment (build 1.8.0-ea-lambda-nightly-h1171-20120911-b56-b00)
OpenJDK 64-Bit Server VM (build 24.0-b21, mixed mode)
$javac -version
javac 1.8.0-ea
If you see the above versions, then you have set the path right and got the libraries working. Write any java 8 lambda program ( click here for sample ) and compile in the same “Terminal” session.
To set the JAVA_HOME permanently, create/open the ~/.bashrc
or ~/.bash_profile
and set the JAVA_HOME path in it.
Compiling & Running Java 8 program
I’ve considered 3 choices here
- Using Terminal
- Using the Eclipse - External Tools features
- Using Apache Ant - Either in Eclipse or Terminal. I prefer Eclipse for a quick start.
Using Terminal
Whatever you did above, are all is what you need to do to run it in terminal. And preferably have it in a shell script.
Using Eclipse - External Tools
All you have to setup is these two items:
javac
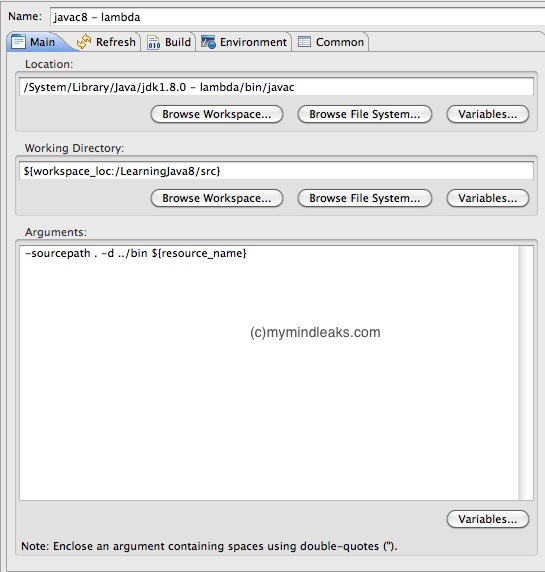
java
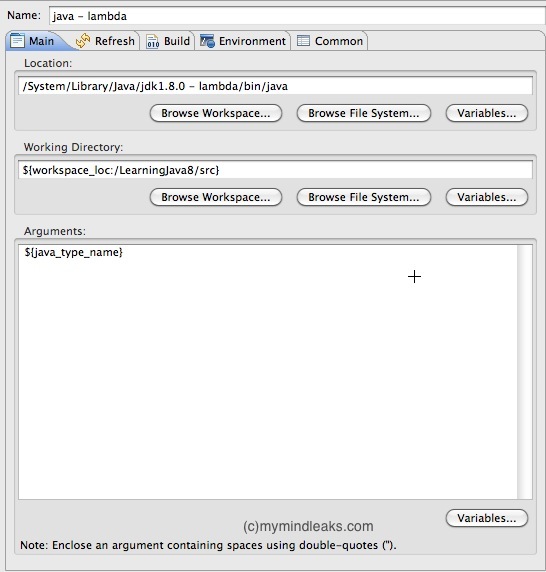
Now run javac and then followed by java.
Using Apache Ant
This is much more customizable and easier to run. Something similar to a Terminal approach, but much nicer.
Here is my an ant script - combines java
& javac
<project name="HelloJava8" default="Main" basedir=".">
<property name="src.dir" location="src" />
<property name="build.dir" location="bin" />
<property name="build.compiler" value="modern" />
<property name="main.class" value="HelloJava8" />
<!-- set java8 as jre -->
<path id="build.classpath"></path>
<!-- Deletes the existing build directory-->
<target name="clean">
<delete dir="${build.dir}" />
</target>
<!-- Creates the build directory-->
<target name="makedir">
<mkdir dir="${build.dir}" />
</target>
<!-- Compiles the java code -->
<target name="compile" depends="clean, makedir">
<javac srcdir="${src.dir}" destdir="${build.dir}" classpathref="build.classpath" />
</target>
<target name="Main" depends="compile">
<description>Main target</description>
<java classname="${main.class}" classpath="${classes.dir};${build.dir}" fork="yes"/>
</target>
</project>
If you are running this in a terminal, create a shell script to export the ANT_HOME
variable to the ANT path.
Running Ant in Eclipse
Make sure to update some settings in Eclipse preferences for Ant and configure the .
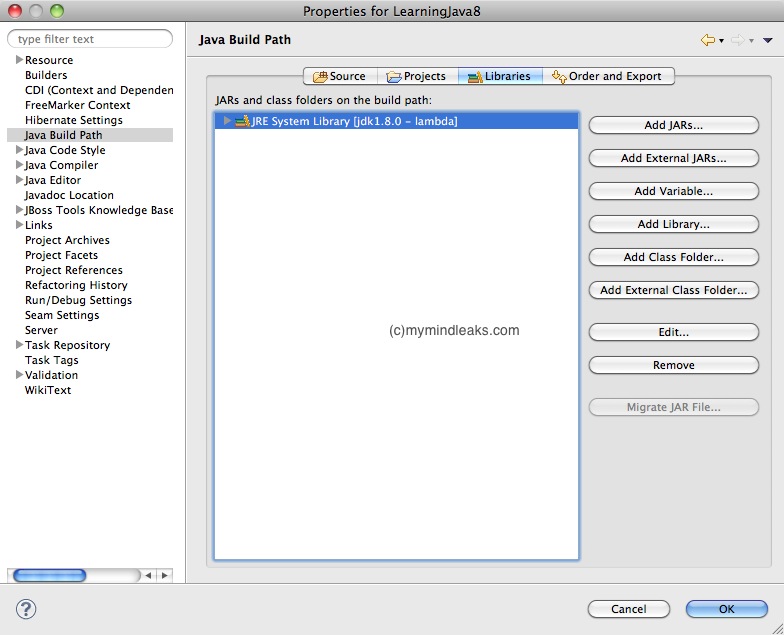
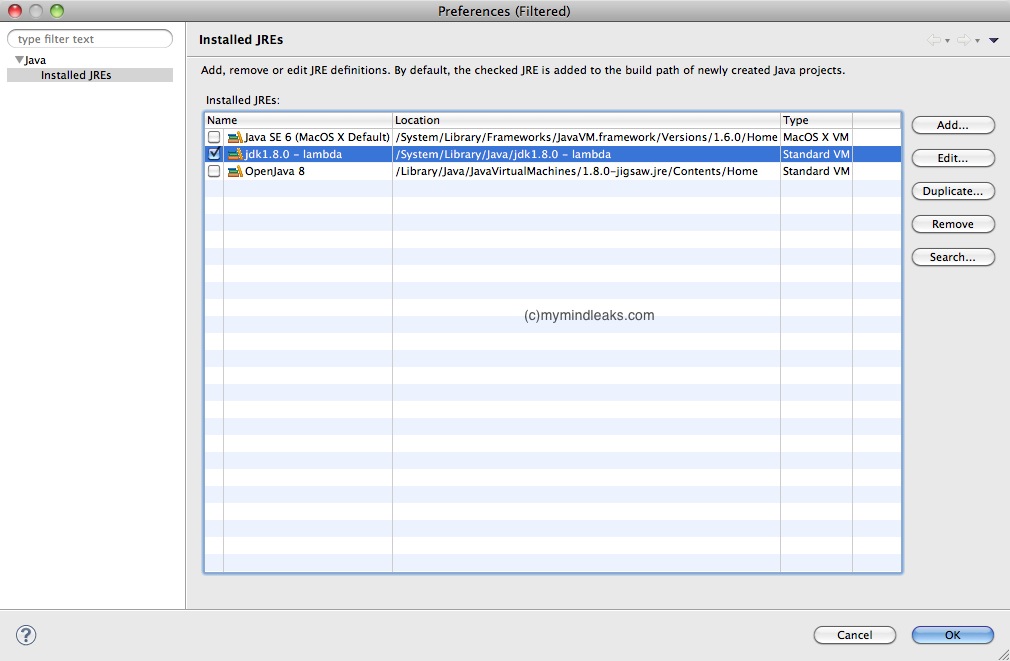
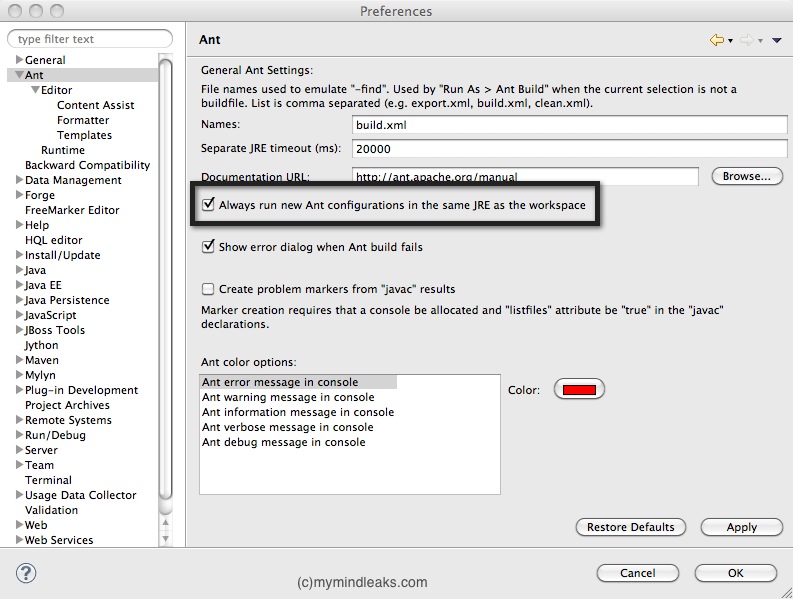
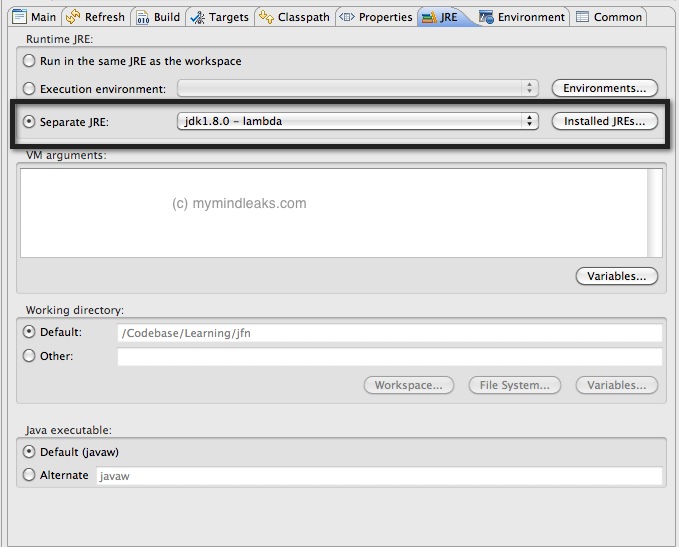
Hello World
And here is a Hello world using a lambda function
to test.
import java.util.Arrays;
import java.util.List;
public class HelloJava8 {
public static void main(String[] args) {
System.out.println("Welcome to Java8! First lambda in java");
List<String> msg = Arrays.asList("So","Nice","that","lambda","works","in", "java");
msg.forEach( e-> { System.out.println(e); } );
}
}